Algorithms and Data Structures
This course covers the fundamental concepts of algorithm design and analysis, as well as the essential data structures used in computing. Students will learn how to analyse and evaluate the performance of algorithms in terms of time and space complexity, and will be introduced to common algorithmic design paradigms such as divide-and-conquer, greedy algorithms, and dynamic programming.
3 Credits
3 weeks
SDE 802
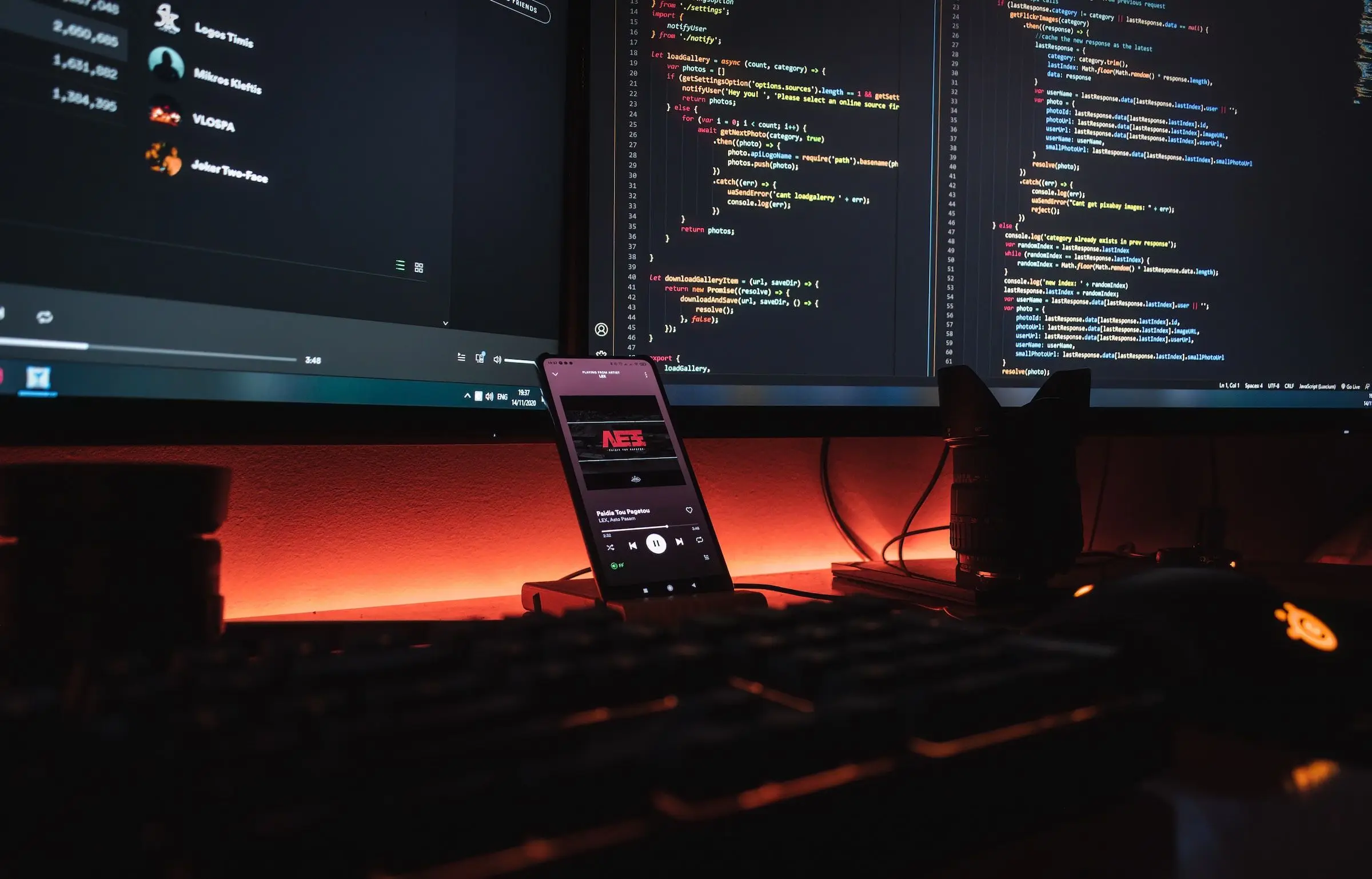
Content
The course covers a range of data structures including arrays, linked lists, stacks, queues, trees, and graphs. Students will learn how to choose the appropriate data structure for a given problem and how to implement them in code.
In addition, the course covers fundamental techniques for searching and sorting data, including linear and binary search, and various sorting algorithms such as bubble sort, merge sort, and quicksort.
Over the duration of this Algorithms and Data Structures course, students will work their way through the following topics:
- Introduction to algorithms and data structures
- Asymptotic analysis and big-O notation
- Sorting algorithms (bubble sort, selection sort, insertion sort, merge sort, quicksort)
- Searching algorithms (linear search, binary search, interpolation search)
- Linked lists and their operations (insertion, deletion, traversal)
- Stacks and queues (using arrays and linked lists)
- Trees (binary trees, traversal, insertion, deletion)
- Binary search trees (BSTs), AVL trees, and red-black trees
- Graphs and graph algorithms (BFS, DFS, shortest path, minimum spanning tree)
- Hash tables and hash functions; dynamic programming
- Greedy algorithms
Outcomes
By the end of the course, students should be able to:
- Analyse and compare different data structures and algorithms in terms of their time and space complexities.
- Design and implement efficient algorithms and data structures for a variety of problems.
- Apply appropriate data structures and algorithms to solve problems in different domains such as computer science, engineering, and natural sciences.
- Evaluate the effectiveness of different algorithmic paradigms, such as divide-and-conquer, dynamic programming, and greedy algorithms.
- Understand the fundamental concepts of computational complexity theory, such as the classes P and NP, and their implications for algorithm design and analysis.
This course is taking under this degree
Apply for this Course